For practicing Java, here are few Core Java Assignments. In Each Assignment, a specific Object Oriented Programming concept is covered. Before start this assignments, make sure you completed all the OOPs Concepts in Java here.
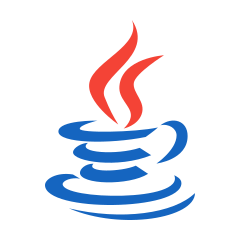
Core Java Assignments 0:
Goal: Learning Class Creation, Object Creation, Method Calling with Arguments, Local Variables
- Create a class called Theatre
- Have main method in it.
- Create an instance[object] called raja of Theatre class.
- Call method bookTicket(200) eg. raja.bookTicket(100)
- Have method signature for bookTicket method with necessary arguments for handling the input 200.
- Define bookTicket method as below.
- Declare a local variable ticket_price in int datatype.
- Assign 120 as value for ticket_price variable.
- Subtract 120 from method argument (200)
- Store result of above line into another variable called balance of int datatype.
- return this balance to main method.
- In main method, receive this balance as int balance
- In main method, print as below.
System.out.println(“After booking ticket ” + balance);
Core Java Assignments 1:
Goal: Learning Method Calling, Accessing static, non-static variables from other classes, private modifier.
Steps:
Create a class Called School.
Have non-static variables as below.
int mark;
private int salary;
Have static variable as below.
static String school_name = "St. Antony's Primary School";
Define non-static methods mentioned below
void conduct_exams()
- Have a print statement inside this method
void publish_results(int mark)
- Have a print statement inside this method to print mark
Now, create another class called Teacher
Create main method inside Teacher class
Create an instance(object) for School class [Object name -> teacher]
Using 'teacher' object, call conduct_exams() method
Using 'teacher' object, call publish_results() method and pass 75 as argument here.
Print school_name
Try to access private variable salary in Teacher class and note down the error message.
Core Java Assignments 2:
Goal: Learning private, default and public Access Modifiers, Creating Package and understanding its usage, Calling Methods with/without arguments.
- Check if you can create private class
- Check if you can create private main method
- Check if you can create Method local variable as private.
Task:
- Create a package called bank.chennai.
- Create a public class called ‘SBI’.
- Have default non-static variables String empName, empId.
- Have default static variable branch_name = ‘chennai’
- Create two default, non-static methods
get_loan(int amount), create_account() with void as return datatype. - Now, in the same package(bank.creditcard), create one more default class called Account_Holder.
- Have main method in this class.
- Try to access all static, non-static variables and non-static methods in SBI class.
- Create another package called bank.madurai.
- In this package, create default class called Account_Holder_Madurai.
- Have main method in this class.
- Try to access all static, non-static variables and non-static methods in SBI class.
- Note down the Errors and rectify those errors and make sure this class gives output without any error.
Core Java Assignments 3:
Goal: Learning Inheritance, Multilevel Inheritance, super keyword
Grandma:
- Create a class called ‘Grandma’.
- Declare Field as below in it.
- String name = “Stella”;
- Have below methods in it.
- public void work()
- Have print statements as you wish in the above methods’ definition.
Mother:
- Create a class called ‘Mother’.
- Declare Field as below in it.
- String name = “Stella”;
- Have below method in it.
- public void work()
- Have print statement to print ‘name’ and super.name in work() definition.
- Add ‘super.work();’ inside work() method.
Kid:
- Create a class called ‘Kid’.
- Declare Field as below in it.
- String name = “Suman”;
- Define main method as ‘public static void main(String[] args)
- Inside main method, create an instance called ‘kid’ for Kid class.
- Have below methods in it.
- public void work()
- public void study()
- Have print statements as you wish in the above two methods’ definition.
- Call study() method from main method.
- Inside study() method, call work() method.
- Print name and super.name inside work() method.
Core Java Assignments 4:
Goal: Learning and Understanding Multilevel Inheritance, Abstraction
- Create an abstract class called HeadOffice.
- Have below normal methods in it. 2.1. public void check_accounts(int amount)
- Have a print statement inside here
2.2. public int pay_tax(int amount) - return this.amount from here
- Have a print statement inside here
- Have an abstract method as below.
3.1. public abstract void receive_Customers() - Create another abstract class called Branch_Plan as sub class of HeadOffice
- Have main method in it.
- Add a print statement inside main method.
- Add below method
- public void do_interview()
- Have a print statement inside here.
- Create another class ‘Branch’ as sub class of Branch_Plan
- Handle abstract methods here with print statements.
- Create an instance called ‘branch’ for Branch class.
- Confirm the below methods can be called.
- public void check_accounts(1000)
- public int pay_tax(2000)
- Check if value is returned.
- public void do_interview()